git clone https://github.com/openliberty/guide-rest-client-reactjs.git
cd guide-rest-client-reactjs
Consuming a RESTful web service with ReactJS
Explore how to access a simple RESTful web service and consume its resources with ReactJS in Open Liberty.
What you’ll learn
You will learn how to access a REST service and deserialize the returned JSON that contains a list of artists and their albums by using an HTTP client with the ReactJS library. You will then present this data by using a ReactJS paginated table component.
ReactJS is a JavaScript library that is used to build user interfaces. Its main purpose is to incorporate a component-based approach to create reusable UI elements. With ReactJS, you can also interface with other libraries and frameworks. Note that the names ReactJS and React are used interchangeably.
The React application in this guide is provided and configured for you in the src/main/frontend
directory. The application uses Next.js, a React-powered framework, to set up the modern React application. The Next.js
framework provides a powerful environment for learning and building React applications, with features like server-side rendering, static site generation, and easy API routes. It is the best way to start building a highly performant React application.
The REST service that provides the resources was written for you in advance in the back end of the application, and it responds with the artists.json
file in the src/resources
directory. You will implement a ReactJS client as the front end of your application, which consumes this JSON file and displays its contents on a single web page.
To learn more about REST services and how you can write them, see the Creating a RESTful web service guide.
Getting started
The fastest way to work through this guide is to clone the Git repository and use the projects that are provided inside:
The start
directory contains the starting project that you will build upon.
The finish
directory contains the finished project that you will build.
Before you begin, make sure you have all the necessary prerequisites.
Try what you’ll build
The finish
directory in the root of this guide contains the finished application. The React front end is already pre-built for you and the static files from the production build can be found in the src/main/webapp/_next/static
directory.
To try out the application, navigate to the finish
directory and run the following Maven goal to build the application and deploy it to Open Liberty:
cd finish
mvn process-resources
mvn liberty:run
After you see the following message, your application Liberty instance is ready:
The defaultServer server is ready to run a smarter planet.
Next, point your browser to the http://localhost:9080 web application root to see the following output:
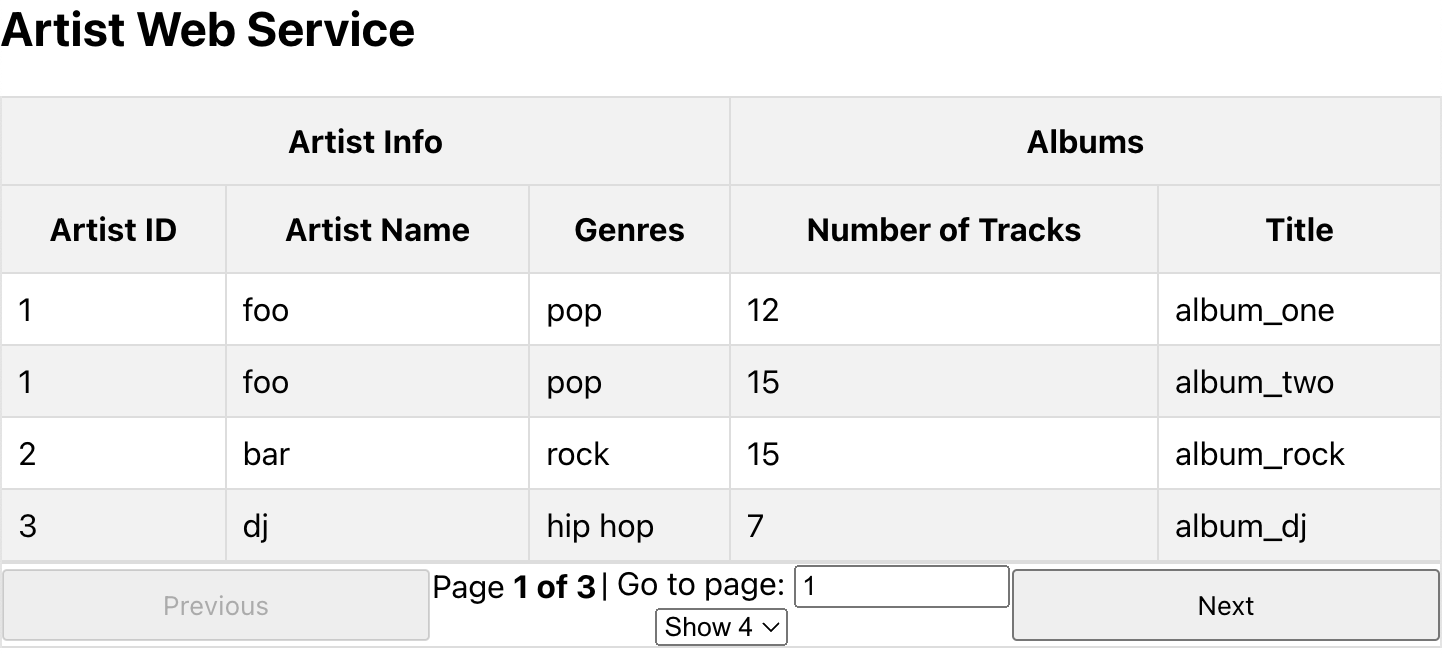
After you are finished checking out the application, stop the Liberty instance by pressing CTRL+C
in the command-line session where you ran Liberty. Alternatively, you can run the liberty:stop
goal from the finish
directory in another shell session:
mvn liberty:stop
Starting the service
Before you begin the implementation, start the provided REST service so that the artist JSON is available to you.
Navigate to the start
directory to begin.
When you run Open Liberty in dev mode, dev mode listens for file changes and automatically recompiles and deploys your updates whenever you save a new change. Run the following goal to start Open Liberty in dev mode:
mvn liberty:dev
After you see the following message, your Liberty instance is ready in dev mode:
************************************************************** * Liberty is running in dev mode.
Dev mode holds your command-line session to listen for file changes. Open another command-line session to continue, or open the project in your editor.
After you start the service, you can find your artist JSON at the http://localhost:9080/artists URL.
All the dependencies for the React front end are listed in the src/main/frontend/src/package.json
file and are installed before the build process by the frontend-maven-plugin
. Also, CSS
stylesheets files are available in the src/main/frontend/src/styles
directory.
Project configuration
The front end of your application uses Node.js to build your React code. The Maven project is configured for you to install Node.js and produce the production files, which are copied to the web content of your application.
Node.js is a server-side JavaScript runtime that is used for developing networking applications. Its convenient package manager, npm, is used to run the React build scripts that are found in the package.json
file. To learn more about Node.js, see the official Node.js documentation.
The frontend-maven-plugin
is used to install
the dependencies that are listed in your package.json
file from the npm registry into a folder called node_modules
. The node_modules
folder can be found in your working
directory. Then, the configuration produces
the production files to the src/main/frontend/build
directory.
The maven-resources-plugin
copies the static
content from the build
directory to the web content
of the application.
Creating the default page
Create the entry point of your React application. The latest version of Next.js
recommends you use the App Router, which centralizes routing logic under the app
directory.
To construct the home page of the web application, create a page.jsx
file.
src/main/frontend/src/app/page.jsx
The page.jsx
file is a container for all other components. When the Home
React component is rendered, the ArtistTable
components content are displayed.
To render the pages correctly, add a layout.jsx
file that defines the RootLayout
containing the UI that are shared across all routes.
src/main/frontend/src/app/layout.jsx
For more detailed information, see the Next.js
documentation on the Layouts and Pages.
Creating the React component
A React web application is a collection of components, and each component has a specific function. You will create a component that the application uses to acquire and display data from the REST API.
Create the ArtistTable
function that fetches data from your back-end and renders it in a table.
src/main/frontend/src/app/ArtistTable.jsx
At the beginning of the file, the use client
directive indicates the ArtistTable
component is rendered on the client side.
The React
library imports the react
package for you to create the ArtistTable
function. This function must have the export
declaration because it is being exported to the page.jsx
module. The posts
object is initialized using a React Hook that lets you add a state to represent the state of the posts that appear on the paginated table.
To display the returned data, you will use pagination. Pagination is the process of separating content into discrete pages, and you can use it for handling data sets in React. In your application, you’ll render the columns in the paginated table. The columns
constant defines the table that is present on the web page.
The useReactTable
hook creates a table instance. The hook takes in the columns
and posts
as parameters. The getCoreRowModel
function is included for the generation of the core row model of the table, which serves as the foundational row model upon pagination and sorting build. The getPaginationRowModel
function applies pagination to the core row model, returning a row model that includes only the rows that should be displayed on the current page based on the pagination state. In addition, the getSortedRowModel
function sorts the paginated table by the column headers then applies the changes to the row model. The paginated table instance is assigned to the table
constant, which renders the paginated table on the web page.
Importing the HTTP client
Your application needs a way to communicate with and retrieve resources from RESTful web services to output the resources onto the paginated table. The Axios library will provide you with an HTTP client. This client is used to make HTTP requests to external resources. Axios is a promise-based HTTP client that can send asynchronous requests to REST endpoints. To learn more about the Axios library and its HTTP client, see the Axios documentation.
The GetArtistsInfo()
function uses the Axios API to fetch data from your back end. This function is called when the ArtistTable
is rendered to the page using the useEffect()
React lifecycle method.
src/main/frontend/src/app/ArtistTable.jsx
Add the axios
library and the GetArtistsInfo()
function.
The axios
HTTP call is used to read the artist JSON that contains the data from the sample JSON file in the resources
directory. When a response is successful, the state of the system changes by assigning response.data
to posts
. The artists
and their albums
JSON data are manipulated to allow them to be accessed by the ReactTable
. The …rest
or …album
object spread syntax is designed for simplicity. To learn more about it, see Spread in object literals.
Building and packaging the front-end
After you successfully build your components, you need to build the front end and package your application. The Maven process-resources
goal generates the Node.js resources, creates the front-end production build, and copies and processes the resources into the destination directory.
In a new command-line session, build the front end by running the following command in the start
directory:
mvn process-resources
The build may take a few minutes to complete. You can rebuild the front end at any time with the Maven process-resources
goal. Any local changes to your JavaScript and HTML are picked up when you build the front-end.
Navigate to the http://localhost:9080 web application root to view the front end of your application.
Testing the React client
Next.js
supports various testing tools. This guide uses Vitest
for unit testing the React components, with the test file App.test.jsx
located in src/main/frontend/tests/
directory. The App.test.jsx
file is a simple JavaScript file that tests against the page.jsx
component. No explicit test cases are written for this application. To learn more about Vitest
, see Setting up Vitest with Next.js.
pom.xml
To run the default test, you can add the testing
configuration to the frontend-maven-plugin
. Rerun the Maven process-resources
goal to rebuild the front end and run the tests.
mvn process-resources
If the test passes, you see a similar output to the following example:
[INFO] ✓ __tests__/App.test.jsx (1 test) 96ms
[INFO]
[INFO] Test Files 1 passed (1)
[INFO] Tests 1 passed (1)
[INFO] Start at 10:43:25
[INFO] Duration 3.73s (transform 264ms, setup 0ms, collect 343ms, tests 96ms, environment 408ms, prepare 1.16s)
Although the React application in this guide is simple, when you build more complex React applications, testing becomes a crucial part of your development lifecycle. If you need to write application-oriented test cases, follow the official React testing documentation.
When you are done checking the application root, exit dev mode by pressing CTRL+C
in the shell session where you ran the Liberty.
1[
2 {
3 "id": "1",
4 "name" : "foo",
5 "genres": "pop",
6 "albums" : [
7 {
8 "title" : "album_one",
9 "artist" : "foo",
10 "ntracks" : 12
11 },
12 {
13 "title" : "album_two",
14 "artist" : "foo",
15 "ntracks" : 15
16 }
17 ]
18 },
19 {
20 "id" : "2",
21 "name" : "bar",
22 "genres": "rock",
23 "albums" : [
24 {
25 "title" : "album_rock",
26 "artist" : "bar",
27 "ntracks" : 15
28 }
29 ]
30 },
31 {
32 "id": "3",
33 "name" : "dj",
34 "genres": "hip hop",
35 "albums" : [
36 {
37 "title" : "album_dj",
38 "artist" : "dj",
39 "ntracks" : 7
40 }
41 ]
42 },
43 {
44 "id": "4",
45 "name" : "flow",
46 "genres": "R&B",
47 "albums" : [
48 {
49 "title" : "album_long",
50 "artist" : "flow",
51 "ntracks" : 22
52 }
53 ]
54 },
55 {
56 "id": "5",
57 "name" : "cc",
58 "genres": "country",
59 "albums" : [
60 {
61 "title" : "album_short",
62 "artist" : "cc",
63 "ntracks" : 5
64 }
65 ]
66 },
67 {
68 "id": "6",
69 "name" : "axis",
70 "genres": "classical",
71 "albums" : [
72 {
73 "title" : "album_one",
74 "artist" : "axis",
75 "ntracks" : 9
76 },
77 {
78 "title" : "album_two",
79 "artist" : "axis",
80 "ntracks" : 8
81 },
82 {
83 "title" : "album_three",
84 "artist" : "axis",
85 "ntracks" : 11
86 }
87 ]
88 }
89]90
Nice work! Where to next?
Nice work! Nice work! You just accessed a simple RESTful web service and consumed its resources by using ReactJS in Open Liberty.
Consuming a RESTful web service with ReactJS by Open Liberty is licensed under CC BY-ND 4.0
What did you think of this guide?




Thank you for your feedback!
What could make this guide better?
Raise an issue to share feedback
Create a pull request to contribute to this guide
Need help?
Ask a question on Stack Overflow